Welcome to Blazor!
The following steps illustrate how to download and install the Syncfusion Blazor Template Studio with the Code Generator extension from the Visual Studio Marketplace. Note: Syncfusion Blazor Code Generator was shipped within the Syncfusion Template Studio. Download the Syncfusion Blazor Template Studio from the Visual Studio Marketplace. Configure your local environment for Blazor development with Visual Studio Code. Create a new Blazor WebAssembly project. Add client-side logic to a Blazor web app. Microsoft.AspNetCore.Razor.VSCode.BlazorWasmDebuggingExtension This is a companion extension to the C# extension that brings some improvements to the experience for debugging Blazor WebAssembly in VS Code. This extension should not be installed by itself and will automatically install the C# extension. For a lightweight code editor, try Visual Studio Code with the C# extension. Visual Studio Code. Develop on Linux, macOS, or Windows to build cross platform websites and services. Install the C# extension to get the best experience. Download Visual Studio Code. Tutorial: Build a Blazor todo list app.
Blazor is a framework for building interactive client-side web UI with .NET:
- Create rich interactive UIs using C# instead of JavaScript.
- Share server-side and client-side app logic written in .NET.
- Render the UI as HTML and CSS for wide browser support, including mobile browsers.
- Integrate with modern hosting platforms, such as Docker.
Using .NET for client-side web development offers the following advantages:
- Write code in C# instead of JavaScript.
- Leverage the existing .NET ecosystem of .NET libraries.
- Share app logic across server and client.
- Benefit from .NET's performance, reliability, and security.
- Stay productive with Visual Studio on Windows, Linux, and macOS.
- Build on a common set of languages, frameworks, and tools that are stable, feature-rich, and easy to use.
Components
Blazor apps are based on components. A component in Blazor is an element of UI, such as a page, dialog, or data entry form.
Components are .NET C# classes built into .NET assemblies that:
- Define flexible UI rendering logic.
- Handle user events.
- Can be nested and reused.
- Can be shared and distributed as Razor class libraries or NuGet packages.
The component class is usually written in the form of a Razor markup page with a .razor
file extension. Components in Blazor are formally referred to as Razor components. Razor is a syntax for combining HTML markup with C# code designed for developer productivity. Razor allows you to switch between HTML markup and C# in the same file with IntelliSense programming support in Visual Studio. Razor Pages and MVC also use Razor. Unlike Razor Pages and MVC, which are built around a request/response model, components are used specifically for client-side UI logic and composition.
Blazor uses natural HTML tags for UI composition. The following Razor markup demonstrates a component (Dialog.razor
) that displays a dialog and processes an event when the user selects a button:
In the preceding example, OnYes
is a C# method triggered by the button's onclick
event. The dialog's text (ChildContent
) and title (Title
) are provided by the following component that uses this component in its UI.
The Dialog
component is nested within another component using an HTML tag. In the following example, the Index
component (Pages/Index.razor
) uses the preceding Dialog
component. The tag's Title
attribute passes a value for the title to the Dialog
component's Title
property. The Dialog
component's text (ChildContent
) are set by the content of the <Dialog>
element. When the Dialog
component is added to the Index
component, IntelliSense in Visual Studio speeds development with syntax and parameter completion.
The dialog is rendered when the Index
component is accessed in a browser. When the button is selected by the user, the browser's developer tools console shows the message written by the OnYes
method:
Components render into an in-memory representation of the browser's Document Object Model (DOM) called a render tree, which is used to update the UI in a flexible and efficient way.
Blazor WebAssembly
Blazor WebAssembly is a single-page app (SPA) framework for building interactive client-side web apps with .NET. Blazor WebAssembly uses open web standards without plugins or recompiling code into other languages. Blazor WebAssembly works in all modern web browsers, including mobile browsers.
Running .NET code inside web browsers is made possible by WebAssembly (abbreviated wasm
). WebAssembly is a compact bytecode format optimized for fast download and maximum execution speed. WebAssembly is an open web standard and supported in web browsers without plugins.
WebAssembly code can access the full functionality of the browser via JavaScript, called JavaScript interoperability, often shortened to JavaScript interop or JS interop. .NET code executed via WebAssembly in the browser runs in the browser's JavaScript sandbox with the protections that the sandbox provides against malicious actions on the client machine.
When a Blazor WebAssembly app is built and run in a browser:
- C# code files and Razor files are compiled into .NET assemblies.
- The assemblies and the .NET runtime are downloaded to the browser.
- Blazor WebAssembly bootstraps the .NET runtime and configures the runtime to load the assemblies for the app. The Blazor WebAssembly runtime uses JavaScript interop to handle DOM manipulation and browser API calls.
Blazer Visual Studio
The size of the published app, its payload size, is a critical performance factor for an app's usability. A large app takes a relatively long time to download to a browser, which diminishes the user experience. Blazor WebAssembly optimizes payload size to reduce download times:
- Unused code is stripped out of the app when it's published by the Intermediate Language (IL) Trimmer.
- HTTP responses are compressed.
- The .NET runtime and assemblies are cached in the browser.
- Unused code is stripped out of the app when it's published by the Intermediate Language (IL) Linker.
- HTTP responses are compressed.
- The .NET runtime and assemblies are cached in the browser.
Blazor Server
Blazor decouples component rendering logic from how UI updates are applied. Blazor Server provides support for hosting Razor components on the server in an ASP.NET Core app. UI updates are handled over a SignalR connection.
The runtime stays on the server and handles:
- Executing the app's C# code.
- Sending UI events from the browser to the server.
- Applying UI updates to the rendered component that are sent back by the server.
The connection used by Blazor Server to communicate with the browser is also used to handle JavaScript interop calls.
JavaScript interop
For apps that require third-party JavaScript libraries and access to browser APIs, components interoperate with JavaScript. Components are capable of using any library or API that JavaScript is able to use. C# code can call into JavaScript code, and JavaScript code can call into C# code.
Code sharing and .NET Standard
Blazor implements the .NET Standard, which enables Blazor projects to reference libraries that conform to .NET Standard specifications. .NET Standard is a formal specification of .NET APIs that are common across .NET implementations. .NET Standard class libraries can be shared across different .NET platforms, such as Blazor, .NET Framework, .NET Core, Xamarin, Mono, and Unity.
APIs that aren't applicable inside of a web browser (for example, accessing the file system, opening a socket, and threading) throw a PlatformNotSupportedException.
Additional resources
- Awesome Blazor community links
This tutorial teaches the basics of building a real-time app using SignalR with Blazor. You learn how to:
- Create a Blazor project
- Add the SignalR client library
- Add a SignalR hub
- Add SignalR services and an endpoint for the SignalR hub
- Add Razor component code for chat
At the end of this tutorial, you'll have a working chat app.
View or download sample code (how to download)
Prerequisites
- Visual Studio 2019 16.8 or later with the ASP.NET and web development workload
The Visual Studio Code instructions use the .NET CLI for ASP.NET Core development functions such as project creation. You can follow these instructions on macOS, Linux, or Windows and with any code editor. Minor changes may be required if you use something other than Visual Studio Code.
- Visual Studio 2019 16.6 or later with the ASP.NET and web development workload
The Visual Studio Code instructions use the .NET Core CLI for ASP.NET Core development functions such as project creation. You can follow these instructions on any platform (macOS, Linux, or Windows) and with any code editor. Minor changes may be required if you use something other than Visual Studio Code. For more information on installing Visual Studio Code on macOS, see Visual Studio Code on macOS.
Create a hosted Blazor WebAssembly app
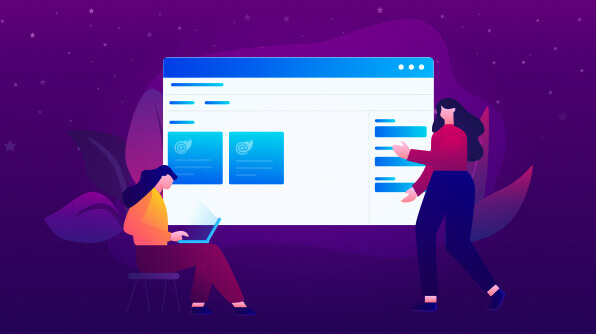
Follow the guidance for your choice of tooling:
Note
Visual Studio 16.8 or later and .NET Core SDK 5.0.0 or later are required.
Note
Visual Studio 16.6 or later and .NET Core SDK 3.1.300 or later are required.
Create a new project.
Select Blazor App and select Next.
Type
BlazorWebAssemblySignalRApp
in the Project name field. Confirm the Location entry is correct or provide a location for the project. Select Create.Choose the Blazor WebAssembly App template.
Under Advanced, select the ASP.NET Core hosted check box.
Select Create.
In a command shell, execute the following command:
The
-ho|--hosted
option creates a hosted Blazor WebAssembly solution. For information on configuring VS Code assets in the.vscode
folder, see the Linux operating system guidance in Tooling for ASP.NET Core Blazor.The
-o|--output
option creates a folder for the solution. If you've created a folder for the solution and the command shell is open in that folder, omit the-o|--output
option and value to create the solution.In Visual Studio Code, open the app's project folder.
When the dialog appears to add assets to build and debug the app, select Yes. Visual Studio Code automatically adds the
.vscode
folder with generatedlaunch.json
andtasks.json
files.
Install the latest version of Visual Studio for Mac and perform the following steps:
Select File > New Solution or create a New project from the Start Window.
In the sidebar, select Web and Console > App.
Choose the Blazor WebAssembly App template. Select Next.
Confirm that Authentication is set to No Authentication. Select the ASP.NET Core Hosted check box. Select Next.
In the Project Name field, name the app
BlazorWebAssemblySignalRApp
. Select Create.If a prompt appears to trust the development certificate, trust the certificate and continue. The user and keychain passwords are required to trust the certificate.
Open the project by navigating to the project folder and opening the project's solution file (
.sln
).
In a command shell, execute the following command:
The -ho|--hosted
option creates a hosted Blazor WebAssembly solution.
The -o|--output
option creates a folder for the solution. If you've created a folder for the solution and the command shell is open in that folder, omit the -o|--output
option and value to create the solution.
Add the SignalR client library
In Solution Explorer, right-click the
BlazorWebAssemblySignalRApp.Client
project and select Manage NuGet Packages.In the Manage NuGet Packages dialog, confirm that the Package source is set to
nuget.org
.With Browse selected, type
Microsoft.AspNetCore.SignalR.Client
in the search box.In the search results, select the
Microsoft.AspNetCore.SignalR.Client
package. Set the version to match the shared framework of the app. Select Install.If the Preview Changes dialog appears, select OK.
If the License Acceptance dialog appears, select I Accept if you agree with the license terms.
In the Integrated Terminal (View > Terminal from the toolbar), execute the following command:
To add an earlier version of the package, supply the --version {VERSION}
option, where the {VERSION}
placeholder is the version of the package to add.
In the Solution sidebar, right-click the
BlazorWebAssemblySignalRApp.Client
project and select Manage NuGet Packages.In the Manage NuGet Packages dialog, confirm that the source drop-down is set to
nuget.org
.With Browse selected, type
Microsoft.AspNetCore.SignalR.Client
in the search box.In the search results, select the check box next to the
Microsoft.AspNetCore.SignalR.Client
package. Set the version to match the shared framework of the app. Select Add Package.If the License Acceptance dialog appears, select Accept if you agree with the license terms.
In a command shell from the solution's folder, execute the following command:
To add an earlier version of the package, supply the --version {VERSION}
option, where the {VERSION}
placeholder is the version of the package to add.
Add a SignalR hub
In the BlazorWebAssemblySignalRApp.Server
project, create a Hubs
(plural) folder and add the following ChatHub
class (Hubs/ChatHub.cs
):
Add services and an endpoint for the SignalR hub
In the
BlazorWebAssemblySignalRApp.Server
project, open theStartup.cs
file.Add the namespace for the
ChatHub
class to the top of the file:
Add SignalR and Response Compression Middleware services to
Startup.ConfigureServices
:In
Startup.Configure
:- Use Response Compression Middleware at the top of the processing pipeline's configuration.
- Between the endpoints for controllers and the client-side fallback, add an endpoint for the hub.
Add SignalR and Response Compression Middleware services to
Startup.ConfigureServices
:In
Startup.Configure
:- Use Response Compression Middleware at the top of the processing pipeline's configuration.
- Between the endpoints for controllers and the client-side fallback, add an endpoint for the hub.
Add Razor component code for chat
- In the
BlazorWebAssemblySignalRApp.Client
project, open thePages/Index.razor
file.
Replace the markup with the following code:
Run the app
Follow the guidance for your tooling:
In Solution Explorer, select the
BlazorWebAssemblySignalRApp.Server
project. Press F5 to run the app with debugging or Ctrl+F5 to run the app without debugging.Copy the URL from the address bar, open another browser instance or tab, and paste the URL in the address bar.
Choose either browser, enter a name and message, and select the button to send the message. The name and message are displayed on both pages instantly:
Quotes: Star Trek VI: The Undiscovered Country ©1991 Paramount
For information on configuring VS Code assets in the .vscode
folder, see the Linux operating system guidance in Tooling for ASP.NET Core Blazor.
Press F5 to run the app with debugging or Ctrl+F5 to run the app without debugging.
Copy the URL from the address bar, open another browser instance or tab, and paste the URL in the address bar.
Choose either browser, enter a name and message, and select the button to send the message. The name and message are displayed on both pages instantly:
Quotes: Star Trek VI: The Undiscovered Country ©1991 Paramount
In the Solution sidebar, select the
BlazorWebAssemblySignalRApp.Server
project. Press ⌘+↩ to run the app with debugging or ⌥+⌘+↩ to run the app without debugging.Copy the URL from the address bar, open another browser instance or tab, and paste the URL in the address bar.
Choose either browser, enter a name and message, and select the button to send the message. The name and message are displayed on both pages instantly:
Quotes: Star Trek VI: The Undiscovered Country ©1991 Paramount
In a command shell from the solution's folder, execute the following commands:
Copy the URL from the address bar, open another browser instance or tab, and paste the URL in the address bar.
Choose either browser, enter a name and message, and select the button to send the message. The name and message are displayed on both pages instantly:
Quotes: Star Trek VI: The Undiscovered Country ©1991 Paramount
Create a Blazor Server app
Follow the guidance for your choice of tooling:
Note
Visual Studio 16.8 or later and .NET Core SDK 5.0.0 or later are required.
Note
Visual Studio 16.6 or later and .NET Core SDK 3.1.300 or later are required.
Create a new project.
Select Blazor App and select Next.
Type
BlazorServerSignalRApp
in the Project name field. Confirm the Location entry is correct or provide a location for the project. Select Create.Choose the Blazor Server App template.
Select Create.
In a command shell, execute the following command:
The
-o|--output
option creates a folder for the project. If you've created a folder for the project and the command shell is open in that folder, omit the-o|--output
option and value to create the project.In Visual Studio Code, open the app's project folder.
When the dialog appears to add assets to build and debug the app, select Yes. Visual Studio Code automatically adds the
.vscode
folder with generatedlaunch.json
andtasks.json
files. For information on configuring VS Code assets in the.vscode
folder, see the Linux operating system guidance in Tooling for ASP.NET Core Blazor.
Install the latest version of Visual Studio for Mac and perform the following steps:
Select File > New Solution or create a New project from the Start Window.
In the sidebar, select Web and Console > App.
Choose the Blazor Server App template. Select Next.
Confirm that Authentication is set to No Authentication. Select Next.
In the Project Name field, name the app
BlazorServerSignalRApp
. Select Create.If a prompt appears to trust the development certificate, trust the certificate and continue. The user and keychain passwords are required to trust the certificate.
Open the project by navigating to the project folder and opening the project's solution file (
.sln
).
In a command shell, execute the following command:
The -o|--output
option creates a folder for the project. If you've created a folder for the project and the command shell is open in that folder, omit the -o|--output
option and value to create the project.
Add the SignalR client library
In Solution Explorer, right-click the
BlazorServerSignalRApp
project and select Manage NuGet Packages.In the Manage NuGet Packages dialog, confirm that the Package source is set to
nuget.org
.With Browse selected, type
Microsoft.AspNetCore.SignalR.Client
in the search box.In the search results, select the
Microsoft.AspNetCore.SignalR.Client
package. Set the version to match the shared framework of the app. Select Install.If the Preview Changes dialog appears, select OK.
If the License Acceptance dialog appears, select I Accept if you agree with the license terms.
In the Integrated Terminal (View > Terminal from the toolbar), execute the following command:
To add an earlier version of the package, supply the --version {VERSION}
option, where the {VERSION}
placeholder is the version of the package to add.
In the Solution sidebar, right-click the
BlazorServerSignalRApp
project and select Manage NuGet Packages.In the Manage NuGet Packages dialog, confirm that the source drop-down is set to
nuget.org
.With Browse selected, type
Microsoft.AspNetCore.SignalR.Client
in the search box.In the search results, select the check box next to the
Microsoft.AspNetCore.SignalR.Client
package. Set the version to match the shared framework of the app. Select Add Package.If the License Acceptance dialog appears, select Accept if you agree with the license terms.
In a command shell from the project's folder, execute the following command:
To add an earlier version of the package, supply the --version {VERSION}
option, where the {VERSION}
placeholder is the version of the package to add.
Add the System.Text.Encodings.Web package
This section only applies to apps for ASP.NET Core version 3.x.
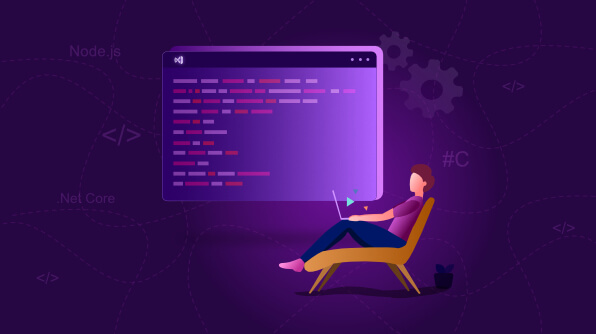
Due to a package resolution issue when using System.Text.Json
5.x in an ASP.NET Core 3.x app, the project requires a package reference for System.Text.Encodings.Web
. The underlying issue will be resolved in a future patch release of .NET 5. For more information, see System.Text.Json defines netcoreapp3.0 with no dependencies (dotnet/runtime #45560).
To add System.Text.Encodings.Web
to the project, follow the guidance for your choice of tooling:

In Solution Explorer, right-click the
BlazorServerSignalRApp
project and select Manage NuGet Packages.In the Manage NuGet Packages dialog, confirm that the Package source is set to
nuget.org
.With Browse selected, type
System.Text.Encodings.Web
in the search box.In the search results, select the
System.Text.Encodings.Web
package. Select the version of the package that matches the shared framework in use. Select Install.If the Preview Changes dialog appears, select OK.
If the License Acceptance dialog appears, select I Accept if you agree with the license terms.
In the Integrated Terminal (View > Terminal from the toolbar), execute the following commands:
To add an earlier version of the package, supply the --version {VERSION}
option, where the {VERSION}
placeholder is the version of the package to add.
In the Solution sidebar, right-click the
BlazorServerSignalRApp
project and select Manage NuGet Packages.In the Manage NuGet Packages dialog, confirm that the source drop-down is set to
nuget.org
.With Browse selected, type
System.Text.Encodings.Web
in the search box.In the search results, select the check box next to the
System.Text.Encodings.Web
package, select the correct version of the package that matches the shared framework in use, and select Add Package.If the License Acceptance dialog appears, select Accept if you agree with the license terms.
In a command shell from the project's folder, execute the following command:
To add an earlier version of the package, supply the --version {VERSION}
option, where the {VERSION}
placeholder is the version of the package to add.
Add a SignalR hub
Create a Hubs
(plural) folder and add the following ChatHub
class (Hubs/ChatHub.cs
):
Add services and an endpoint for the SignalR hub
Open the
Startup.cs
file.Add the namespaces for Microsoft.AspNetCore.ResponseCompression and the
ChatHub
class to the top of the file:
Visual Studio Code Blazor Wasm
Add Response Compression Middleware services to
Startup.ConfigureServices
:In
Startup.Configure
:- Use Response Compression Middleware at the top of the processing pipeline's configuration.
- Between the endpoints for mapping the Blazor hub and the client-side fallback, add an endpoint for the hub.
Add Response Compression Middleware services to
Startup.ConfigureServices
:In
Startup.Configure
:- Use Response Compression Middleware at the top of the processing pipeline's configuration.
- Between the endpoints for mapping the Blazor hub and the client-side fallback, add an endpoint for the hub.
Add Razor component code for chat
- Open the
Pages/Index.razor
file.
Replace the markup with the following code:
Run the app
Follow the guidance for your tooling:
Press F5 to run the app with debugging or Ctrl+F5 to run the app without debugging.
Copy the URL from the address bar, open another browser instance or tab, and paste the URL in the address bar.
Choose either browser, enter a name and message, and select the button to send the message. The name and message are displayed on both pages instantly:
Quotes: Star Trek VI: The Undiscovered Country ©1991 Paramount
Press F5 to run the app with debugging or Ctrl+F5 to run the app without debugging.
Copy the URL from the address bar, open another browser instance or tab, and paste the URL in the address bar.
Choose either browser, enter a name and message, and select the button to send the message. The name and message are displayed on both pages instantly:
Quotes: Star Trek VI: The Undiscovered Country ©1991 Paramount
Press ⌘+↩ to run the app with debugging or ⌥+⌘+↩ to run the app without debugging.
Copy the URL from the address bar, open another browser instance or tab, and paste the URL in the address bar.
Choose either browser, enter a name and message, and select the button to send the message. The name and message are displayed on both pages instantly:
Quotes: Star Trek VI: The Undiscovered Country ©1991 Paramount
In a command shell from the project's folder, execute the following commands:
Copy the URL from the address bar, open another browser instance or tab, and paste the URL in the address bar.
Choose either browser, enter a name and message, and select the button to send the message. The name and message are displayed on both pages instantly:
Quotes: Star Trek VI: The Undiscovered Country ©1991 Paramount
Next steps
In this tutorial, you learned how to:
- Create a Blazor project
- Add the SignalR client library
- Add a SignalR hub
- Add SignalR services and an endpoint for the SignalR hub
- Add Razor component code for chat
To learn more about building Blazor apps, see the Blazor documentation:
Additional resources
